React Query is a powerful and convenient library for managing server-side data in React projects. With its ability to store data in cache, synchronize it, and manage API states, React Query is increasingly being adopted in modern web applications. Let’s explore the key concepts and basic usage of this essential library.
What is React Query?
React Query simplifies data handling from servers, ensuring efficient synchronization and management of API states (such as loading, error, success, etc.). Here are the core concepts you need to know when working with this library:
Key Concepts of React Query
- Query: Used to fetch data from a server or another data source. Queries in React Query are defined using the
useQuery
hook, where you provide a key to identify the query and a fetcher function to retrieve the data. - Mutation: Used to change data, such as adding, updating, or deleting records. It is implemented using the
useMutation
hook. - Cache: automatically caches the data to enhance performance and reduce the number of requests sent to the server.
- Query Invalidation: When data changes, it’s essential to refresh relevant queries to update the user interface. This library provides methods to invalidate and refresh queries as data changes.
- Background Fetching: supports background fetching, ensuring that data is always up to date without interrupting the user experience.
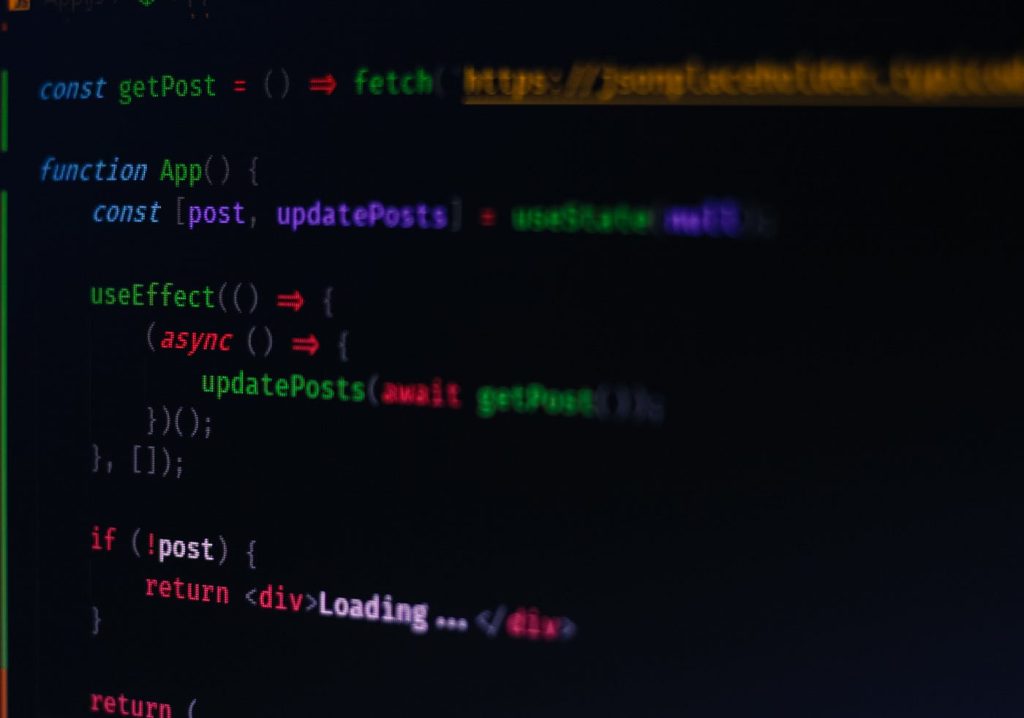
How to Use React Query in Your Project
Setting up in a project is simple. Below is a basic guide to get started.
1. Declaration
javascriptCopy codeimport React from 'react';
import { QueryClientProvider, QueryClient } from 'react-query';
import Main from './Main';
// QueryClient is the central configuration for React Query
const queryClient = new QueryClient({
defaultOptions: {
queries: {
retry: 0,
cacheTime: 0,
refetchOnWindowFocus: false,
},
},
});
function App() {
return (
<QueryClientProvider client={queryClient}>
<Main />
</QueryClientProvider>
);
}
export default App;
Here, QueryClientProvider
wraps the entire application and provides access to queries and mutations.
2. Using useQuery
The useQuery
hook is used to fetch data from a server and manage the different states (loading, error, success) of that data.
javascriptCopy codeimport { useQuery } from 'react-query';
function Example() {
const { isPending, error, data } = useQuery({
queryKey: ['repoData'],
queryFn: () => fetch('https://example.com').then(res => res.json()),
});
if (isPending) return 'Loading...';
if (error) return `An error has occurred: ${error.message}`;
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>{data.subscribers_count}</strong>
<strong>{data.stargazers_count}</strong>
<strong>{data.forks_count}</strong>
</div>
);
}
In this example, the query fetches data from an API and handles the loading, error, and success states.
3. Using useMutation
The useMutation
hook is useful for performing actions that modify data, such as creating, updating, or deleting records.
javascriptCopy codeimport React from 'react';
import { useMutation } from 'react-query';
function SubmitButton() {
const mutation = useMutation(data =>
fetch('https://example.com/api/submit', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
}), {
onSuccess: () => alert('Data submitted successfully!'),
onError: error => alert(`Error: ${error.message}`),
}
);
const handleSubmit = () => {
mutation.mutate({ value: '' });
};
return (
<div>
<button onClick={handleSubmit} disabled={mutation.isLoading}>
{mutation.isLoading ? 'Submitting...' : 'Submit'}
</button>
{mutation.isError && <div>Error: {mutation.error.message}</div>}
{mutation.isSuccess && <div>Data submitted successfully!</div>}
</div>
);
}
export default SubmitButton;
In this example, the mutation submits data to the server and handles success and error states.
Conclusion
React Query is a versatile tool that greatly simplifies data management in applications. With features like caching, background fetching, and query invalidation, it allows developers to create seamless and efficient data-driven apps. For more detailed information on advanced features such as revalidation and cache management, refer to this documentation.